Define AWS Infrastructure in Python with AWS Cloud Development Kit
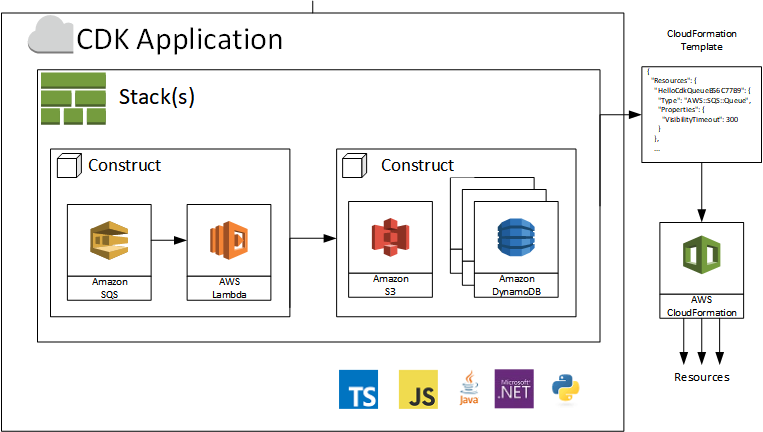
With AWS CDK, we can define an infrastructure using familiar languages (Python, JS, Java, etc) . The code will be compiled into a cloud formation JSON and deployed as a cloud formation stack. Later we can diff the changes made before redeploy.
Install
brew install node
npm install -g aws-cdk
Initialize
cdk init app --language python
python3 -m venv .venv
source .venv/bin/activate
python -m pip install -r requirements.txt
pip install aws-cdk.aws-s3 # module to manage s3
Define
Edit {project_name}/{project_name}_stack.py
from aws_cdk import core
from aws_cdk import aws_s3 as s3
class FnInfraStack(core.Stack):
def __init__(self, scope: core.Construct, construct_id: str, **kwargs) -> None:
super().__init__(scope, construct_id, **kwargs)
s3.bucket(self, "TestingBucketVarokas", versioned=True, )
Preview the cloud formation template with
cdk synth
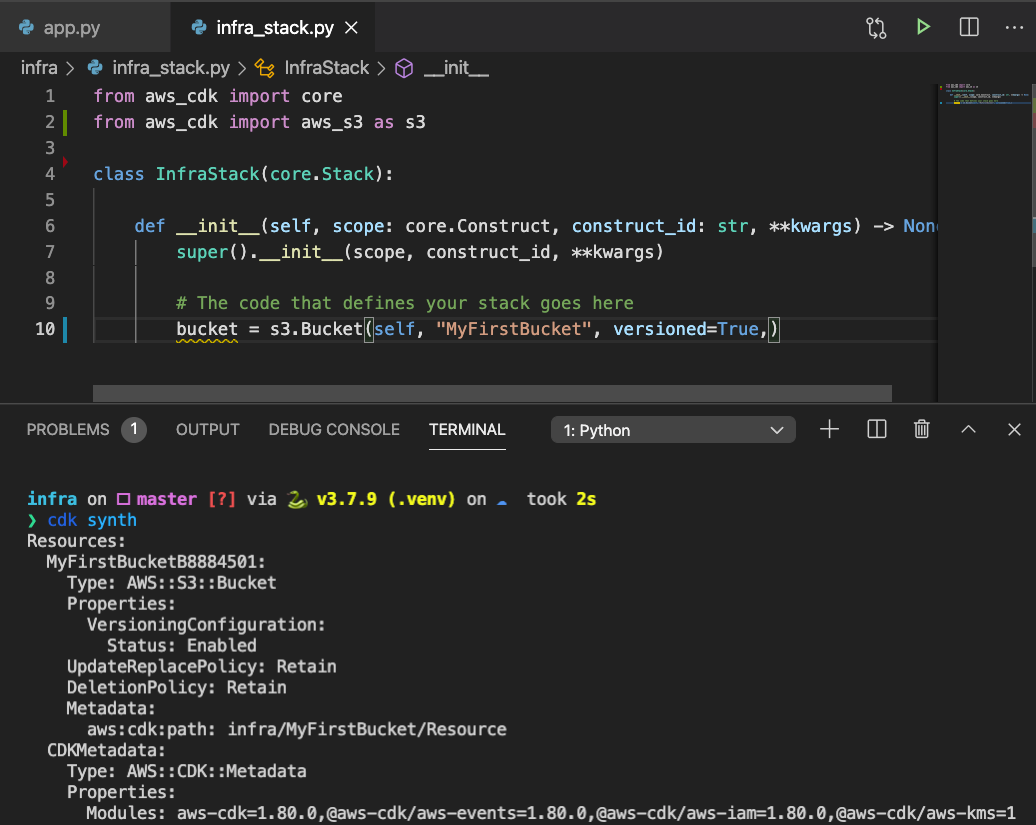
Deploy
We can then immediately deploy the changes as CloudFormation stack
cdk --profile varokas deploy
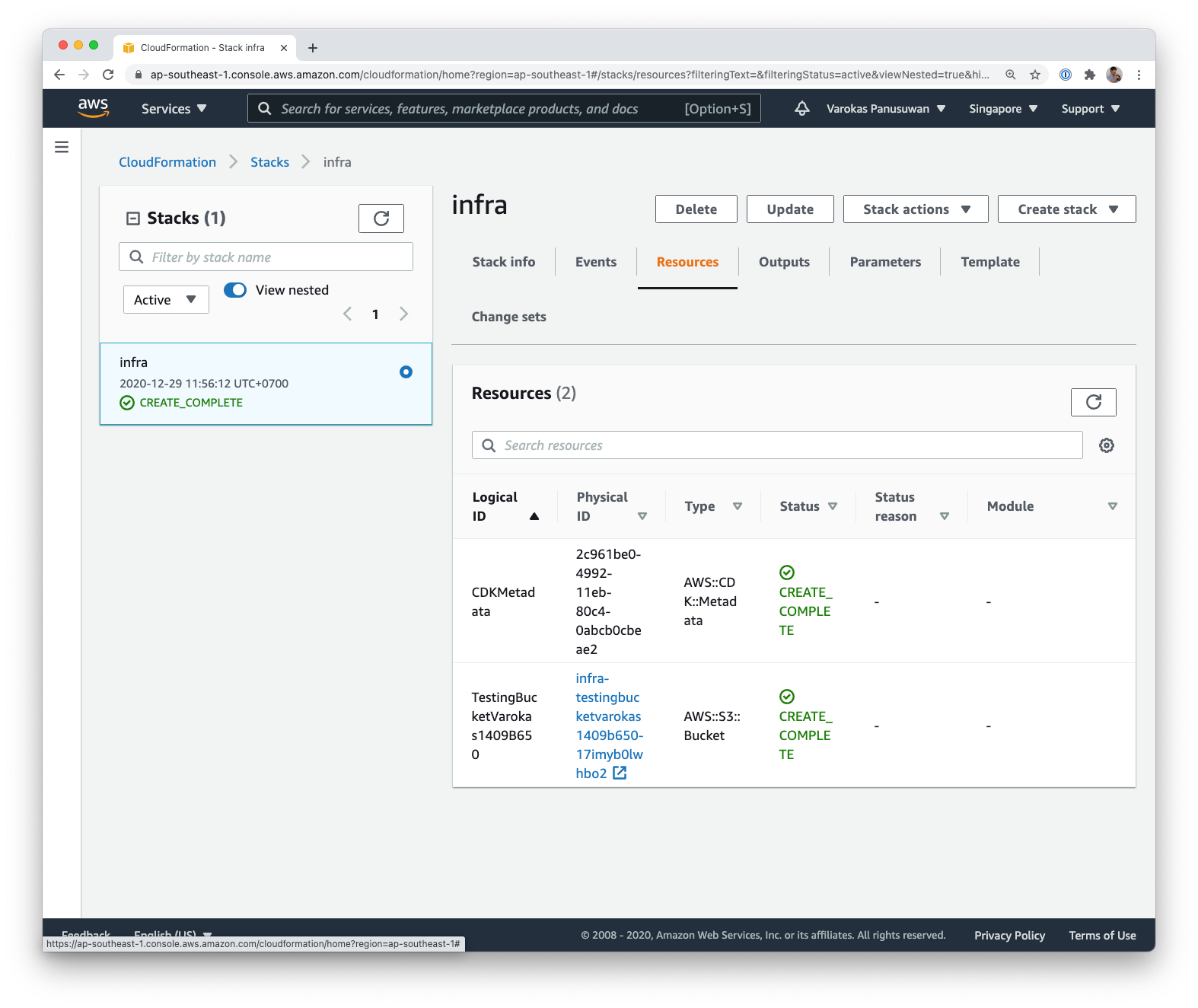
Changes and Diffs
Any changes to the code can be diff-ed against currently deployed stack
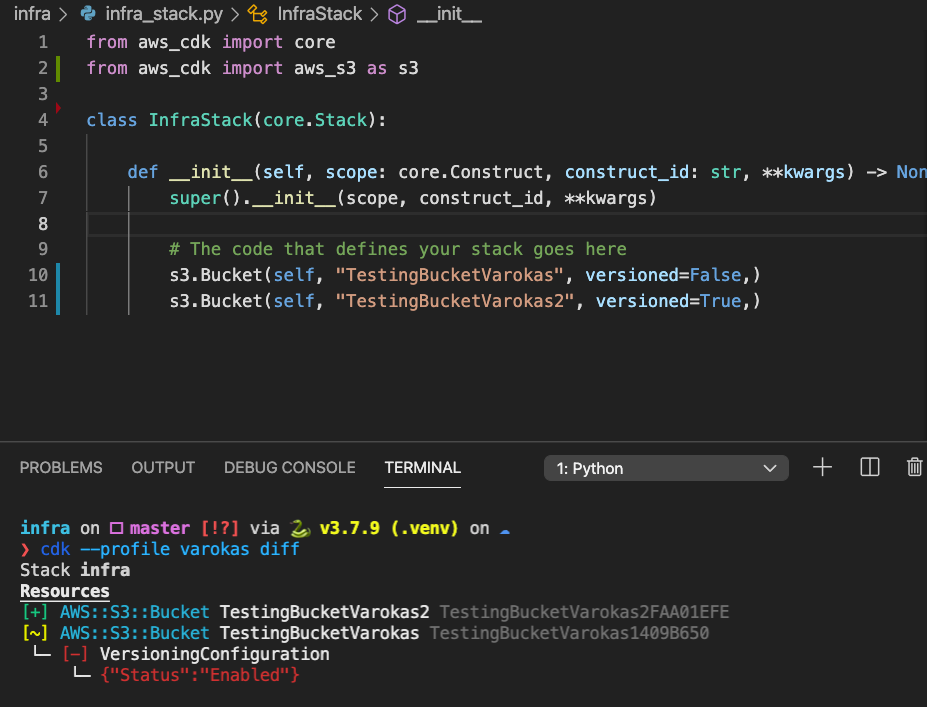
Limitations
There isn't a way to import existing AWS resources to CDK yet
Support importing existing resources into a stack · Issue #84 · aws/aws-cdk-rfcs
Importing existing resources into a CloudFormation stack is now supported! 🎉 The CDK should provide a elegant way to support this. https://aws.amazon.com/blogs/aws/new-import-existing-resources-int...
A workaround exist by creating a failed CloudFormation deployment and fix the template.
How to import existing AWS resources into CDK stack
If you are not creating your account from scratch at the same time when you’re starting to work with CDK, you might need to import existing resources to CDK stack to be able to manage them. This is…
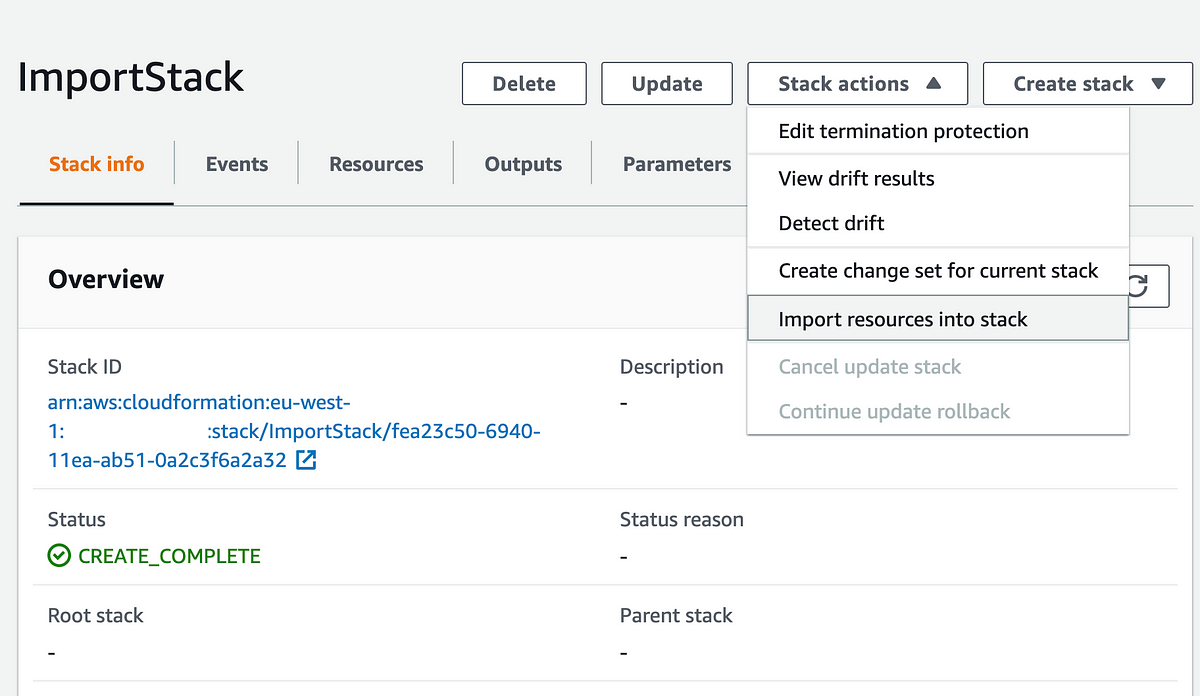
References
Getting started with the AWS CDK - AWS Cloud Development Kit (AWS CDK)
This topic introduces you to important AWS CDK concepts and describes how to install and configure the AWS CDK.